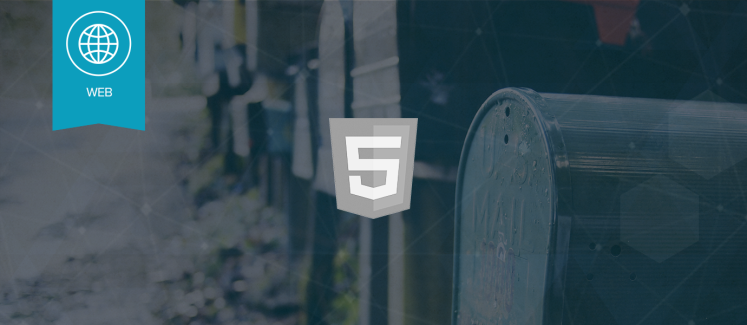
When we talk about web technologies, it’s rare when HTML doesn’t come up. It’s the core language of nearly all web content. Most of what you see on screen in your browser is described, fundamentally, using HTML. More precisely, HTML is the language that describes the structure and the semantic content of a web document.
HTML5 is the latest evolution of the standard that defines HTML. The term represents two different concepts:
- It is a new version of the language HTML, with new elements, attributes, and behaviors,
- a larger set of technologies that allows more diverse and powerful web sites and applications. This set is sometimes called HTML5 & friends and often shortened to just HTML5.
You can find more info about HTML5 Clicking here.
HTML5 Notifications in the Browser
Nowadays, we are seeing a change in the behavior of desktop/mobile websites. Websites are starting to emulate app-like behavior; one of them primarily being notifications. Users have multiple tabs open their browser and they get notifications inside the browser itself, like a chat message, a tweet or Facebook notification.
In this tutorial, we’ll show you how to integrate HTML5s Notification API to send and receive real-time notifications and alerts in the browser. You can learn more about Notification API methods and support from Notification API docs (MDN).
According to Web workers API docs in MDN, this is how HTML5 Notification API works:
“ServiceWorkers essentially act as proxy servers that sit between web applications, and the browser and network (when available). They are intended to (amongst other things) enable the creation of effective offline experiences, intercepting network requests and taking appropriate action based on whether the network is available and updated assets reside on the server. They will also allow access to push notifications and background sync APIs.”
How It Works
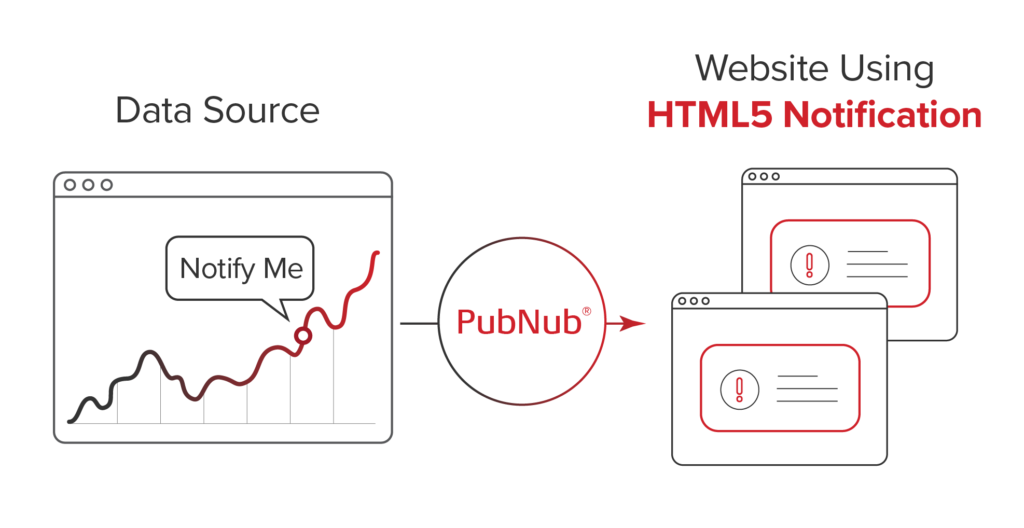
You can find the source code for this tutorial Clicking here.
Index.html
It begins with a basic HTML5 boilerplate with CDN for jQuery and PubNub.
<script src="https://cdn.pubnub.com/pubnub-3.7.13.min.js"></script> <script src="https://code.jquery.com/jquery-1.12.0.min.js"></script>
Then, inside the body tag, we added a button calling a JavaScript function to test the Notification functionality.
<button onclick="notifyMe()">Notify me!</button>
Here is how the notifyMe function looks like:
function notifyMe(message) { if (message == undefined) { message = "Hi there! You clicked a button."; };
Here it checks if the function is getting any parameters. If not, we are setting a message as on clicking the button no message is being passed.
if (!("Notification" in window)) { alert("This browser does not support desktop notification"); } else if (Notification.permission === "granted") { var notification = new Notification(message); } else if (Notification.permission !== 'denied') { Notification.requestPermission(function (permission) { if (permission === "granted") { var notification = new Notification("Hi there!"); } }); }
This block checks if the browser supports notifications. If it supports, then we check whether notification permissions have already been granted. If not, we need to ask the user for permission. If they allow, then we are good to go to create notifications and we pass the message parameters to the notification.
So we have a basic our HTML5 notification API working. Now we need to get the messages from PubNub’s channel and pass it to notification API to make these notifications really real-time.
$(document).ready(function() { var pubnub = PUBNUB({ subscribe_key : 'demo' }); pubnub.subscribe({ channel : "pubnub-html5-notification-demo", // Subscribing to PubNub's channel message : function(message){ console.log(message); notifyMe(message.text); } }) });
Firstly, as soon as the document or HTML page is loaded, we are initializing the PubNub using before mentioned CDN. We instantiate with a subscribe key as only subscribe_key is needed if there is no publishing to channel. Then we are telling to subscribe to “pubnub-html5-notification-demo” channel, and whatever message comes through it, pass it to notifyMe function as parameter.
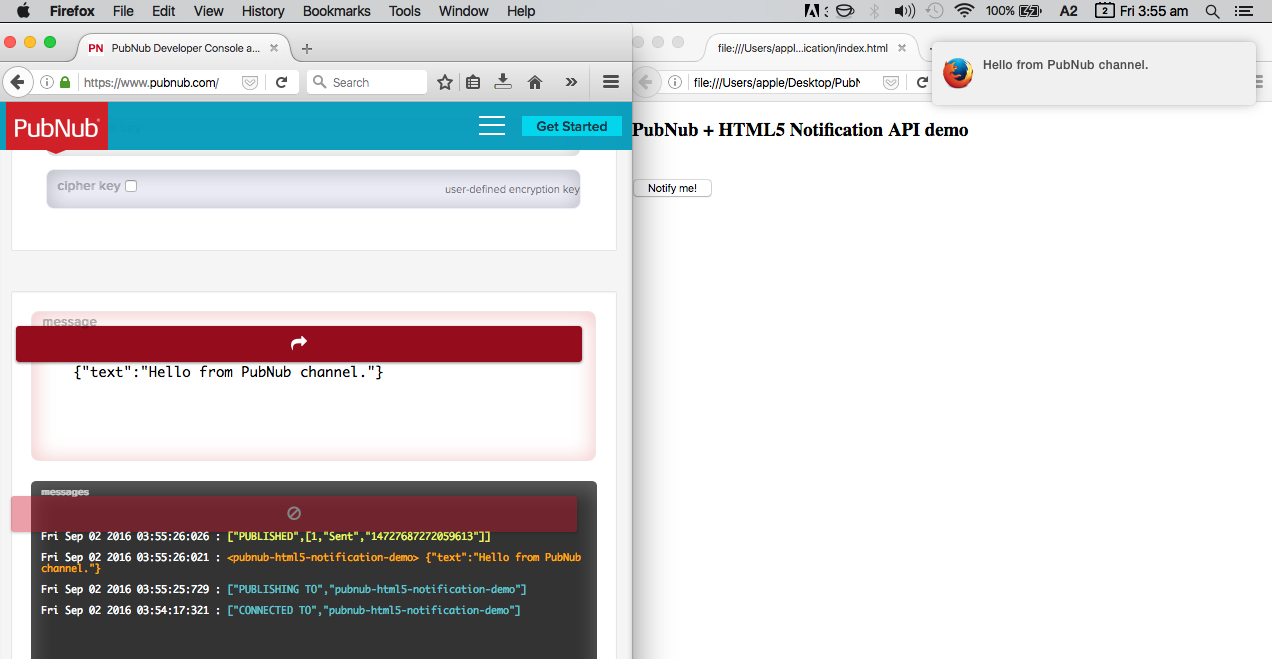
Conclusion
With HTML5 support new features like access to Device APIs, Semantics, Offline and Storage and Multimedia, it’s clear that it is here to stay. One place where you can already see it getting used extensively is mobile website of Facebook. Even when you are browsing it and you get a new notification, it shows up on the screen without page refresh and that’s possible using HTML5’s Notification API.